Table of Contents
Related Posts
Hello World đź‘‹
Welcome to the third article of the My Review of Kent C. Dodds’s EpicReact.Dev Series which is based on the workshop material from EpicReact.Dev by Kent C. Dodds. If you haven’t read the previous articles of the series yet, please go and read them before continuing through this article.
Here are the previous articles.
In this article, You are going to learn the very basics of React. We will just be working with basic HTML and javascript using React raw APIs. We will not even be using any JSX in this article(If you don’t know what JSX is, don’t worry, we will learn about it in the next article.) You will see why it is difficult to work with React raw APIs. Many people skip over these fundamentals before learning React, but it is important to know about these abstractions to understand some things in React which we will see in the next article.
We will follow the similar format of the workshop - meaning for every topic, we will first introduce what we are trying to achieve, then we will see how to achieve that.
Â
Hello World 👋Basic JS “Hello World”IntroductionGenerate DOM NodesIntro to Raw React APIsIntroduction 2Raw React APIsNesting ElementsWhat’s NextUntil Next Time 👋
Basic JS “Hello World”
Introduction
Our goal is to render
Hello World
using basic javascript.Our HTML currently has the following
<div id="root"></div>
We want our HTML to be:
<div id="root">
<div class="container">Hello World</div>
</div>
Generate DOM Nodes
We can achieve the above result by making use of Javascript’s
document
API.// Fetches the element with id as `root` from DOM
const rootElement = document.getElementById("root")
// Creates an element with `div` tag
const helloWorldElement = document.createElement("div")
helloWorldElement.textContent = "Hello World"
helloWorldElement.className = "container"
// Appends the helloWorldElement to the rootElement
rootElement.append(helloWorldElement)
Let’s break down what we are doing here. 1. Get the element with
id
as root
from DOM. 1. Create a new DOM element and then set its properties. 1. Append the newly created element to the root element that we fetched from DOM.Even though the above code is very clear, I broke it down into steps, because in the following section, we will use these exact steps to achieve this, but by using React APIs.
Intro to Raw React APIs
Introduction 2
React uses the same document API that we have seen before under the hood. But it abstracts it away and gives you easy to use and intuitive API to work with
Let’s try to create the same hello world markup that we did before, this time using React.
Raw React APIs
// Fetches the element with id as `root` from DOM
const rootElement = document.getElementById("root")
// Creates an element with `div` tag
const helloWorldElement = React.createElement("div", {
className: "container",
children: "Hello World"
})
// Appends the helloWorldElement to the rootElement
ReactDOM.render(helloWorldElement, rootElement)
Before we understand this code, observe that we have used
React
and ReactDOM
, which are not a part of basic javascript. Hence, they should be added before they become available to us.Let’s add them using their CDN scripts. We will be using unpkg’s CDN.
<script src="https://unpkg.com/react@16.7.0/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.7.0/umd/react-dom.production.min.js"></script>
After adding this, we will be able to access
React
and ReactDOM
from within javascript.Let’s try to understand the code now. 1. Get the element with
id
as root
from DOM. - This part did not change. We are still using the document
API to get the root element. 1. Create a new DOM element and then set its properties. - Here we are introducing the new React API called React.createElement()
. - It takes two arguments 1. HTML tag that we want to create. 1. Properties and the corresponding values that we want to set. - Note that we have introduced a new property called children
. - children
is basically a replacement of what we want inside of the HTML tag that we create. - So, if we want to render <div>Hello World</div>
, we will create a React element with a div
tag and set the children’s property to Hello World
. 1. Append the newly created element to the root element that we fetched from DOM. - We will be using ReactDOM for rendering. The corresponding API is ReactDOM.render()
- It also takes two arguments. - Element that we want to append. - Element which we want to append the above element to. - So, if we want to append element1
to element2
. You would do ReactDOM.render(element1, element2)
.Can you see and appreciate how similar both the React APIs and document APIs are. With the knowledge that we have let’s try to create the below markup using React.
Nesting Elements
Lets try to create the following markup with React.
<div id="root">
<div>
<span>Hello</span>
<span>World</span>
</div>
</div>
Before doing this, you need to know that the
children
property that we have seen before will also accept an array as its value. For example, both of the following calls will produce the same HTML output.// 1.
React.createElement("div", { children: "Hello World" })
// 2.
React.createElement("div", { children: ["Hello", " ", "World"] })
Now that we know this, let’s try to create the given markup.
// Fetches the element with id as `root`
const rootElement = document.getElementById("root")
// Creates a `span` element with content as `Hello`
const helloElement = React.createElement("span", {children: "Hello"})
// Creates a `span` element with content as `World`
const worldElement = React.createElement("span", {children: "World"})
// Let's put the above two elements in to a single div
const helloWorldElement = React.createElement("div", {
children: [helloElement, worldElement]
})
The above code will create the HTML markup that we want.
Note:
React.createElement can also take more than 2 arguments. The following two calls generate the same thing.
// 1.
React.createElement("div", {children: [element1, element2, element3]})
// 2.
React.createElement("div", {}, element1, element2, element3)
So, you can essentially unpack the
children
array and then add them as other arguments.That’s it for now.
What’s Next
In this article, you saw how verbose React raw APIs are. So, it becomes a little difficult to write code like this when there are a lot of elements and each element has different properties.
That is why React gave us a way to simplify this and write the code in a format called
JSX
which looks a bit similar to HTML
. In the next article, we will see all about JSX
and we will also see how to create custom components and style them with CSS.Until Next Time đź‘‹
Links and References:
- EpicReact.Dev - Series of workshops by Kent C. Dodds based on which this blog post series is being written.
- React Fundamentals Workshop Repo - Github Repo if you want to do the self-paced workshop yourself.
- React Fundamentals Workshop Demo - Production application of the above workshop repo.
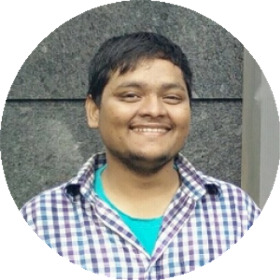
Written by
24yo developer and blogger. I quit my software dev job to make it as an independent maker. I write about bootsrapping Feather.